给你一个整数数组 nums
,找出并返回所有该数组中不同的递增子序列,递增子序列中 至少有两个元素 。你可以按 任意顺序 返回答案。
数组中可能含有重复元素,如出现两个整数相等,也可以视作递增序列的一种特殊情况。
示例 1:
示例 2:
输入:nums = [4,4,3,2,1] 输出:[[4,4]]
|
提示:
1 <= nums.length <= 15
-100 <= nums[i] <= 100
C++
class Solution { public: vector<vector<int>> res; vector<int> path; vector<vector<int>> findSubsequences(vector<int>& nums) { dfs(nums, 0); return res; }
void dfs(vector<int>& nums, int idx) { if(path.size() >= 2 || idx == nums.size()){ if(path.size() >= 2) res.push_back(path); return; }
for(int i = idx; i < nums.size(); i ++) { if(path.size() == 0 || path.back() <= nums[i]){ path.push_back(nums[i]); dfs(nums, i + 1); path.pop_back(); } } } };
|
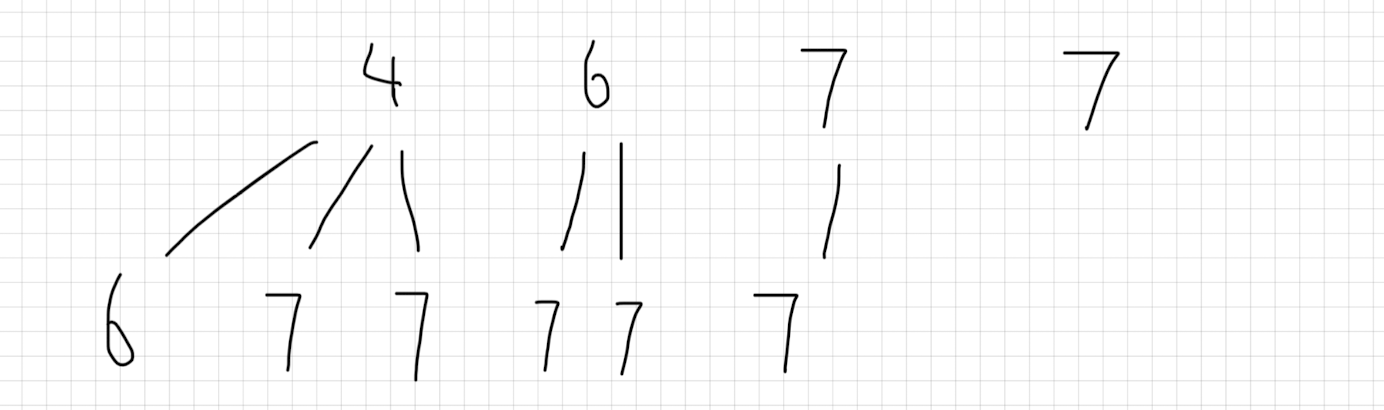
class Solution { public: vector<vector<int>> res; vector<int> path; set<vector<int>> st; vector<vector<int>> findSubsequences(vector<int>& nums) { dfs(nums, 0); return res; }
void dfs(vector<int>& nums, int idx) { if(idx == nums.size()){ if(path.size() >= 2 && st.find(path) == st.end() ){ res.push_back(path); st.insert(path); } return; } dfs(nums, idx + 1);
if(path.size() == 0 || path.back() <= nums[idx]) { path.push_back(nums[idx]); dfs(nums, idx + 1); path.pop_back(); } } };
|
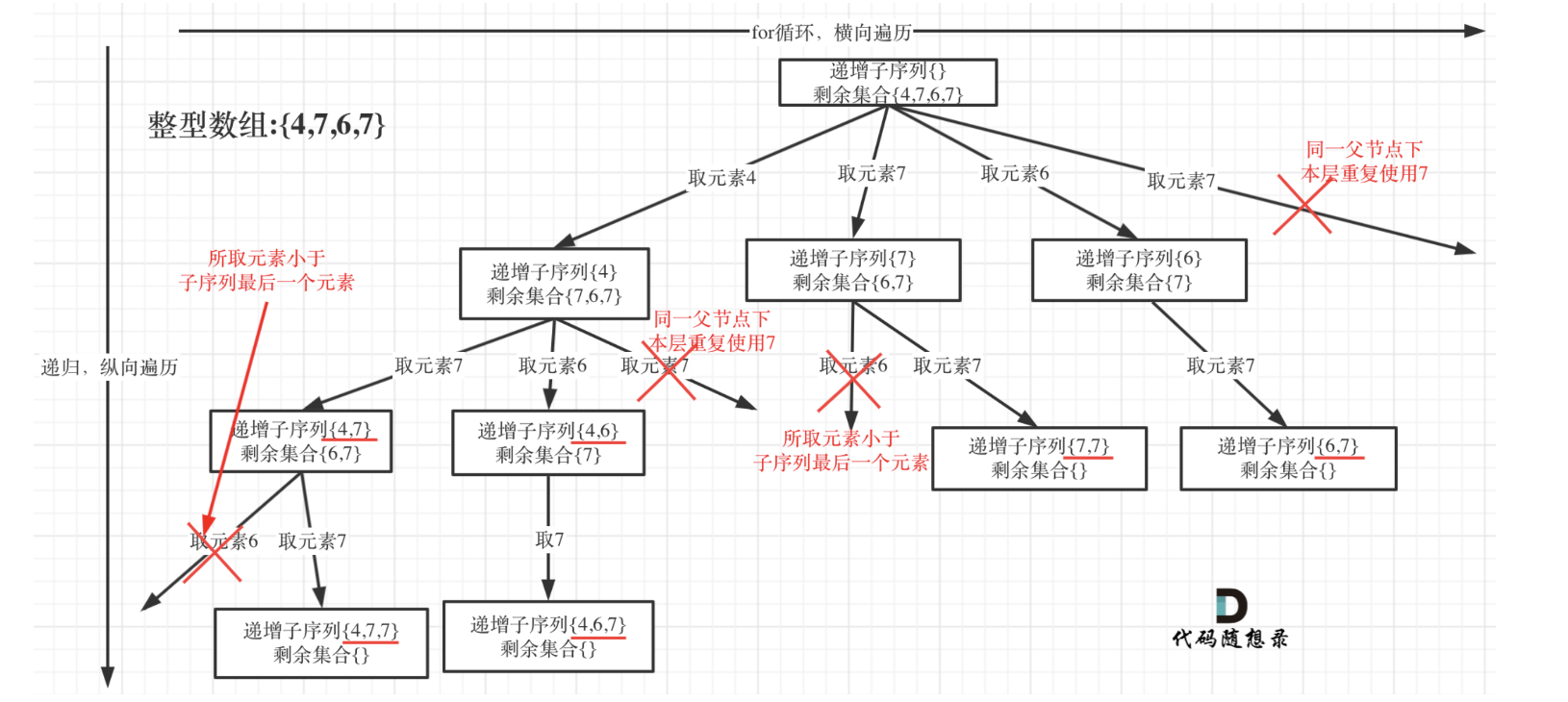
class Solution { private: vector<vector<int>> result; vector<int> path; void backtracking(vector<int>& nums, int startIndex) { if (path.size() > 1) { result.push_back(path); } unordered_set<int> uset; for (int i = startIndex; i < nums.size(); i++) { if ((!path.empty() && nums[i] < path.back()) || uset.find(nums[i]) != uset.end()) continue; uset.insert(nums[i]); path.push_back(nums[i]); backtracking(nums, i + 1); path.pop_back(); } } public: vector<vector<int>> findSubsequences(vector<int>& nums) { backtracking(nums, 0); return result; } };
|